Software Design pattern
For
Real Time Example
- we can say that a design pattern is general reusable solution to a commonly occurring problem within a given context in software design.
- It is not a finished design that can directly transformed into source or machine code.It is description or template for how to solve a problem that can be transformed directly into source or machine code.
- Design pattern are part of the cutting edge of object-oriented technology.Object-oriented analysis tool,books and seminar are incorporating design pattern.
- Object-oriented design pattern typically show relationship and interaction between classes or objects that are involved.
Design pattern are solution to the general problem that software developer faces during software development.
These solution were obtained by trial and error by numerous software developer over quite a sustainable period of time.
*@ LIST AND CLASSIFICATION OF DESIGN PATTERN @*
Design pattern were originally grouped into three categories:
1. Creational patterns
2. Structural patterns
3. Behavioral patterns
All the pattern fall into these three categories. Some of them are listed below as shown:-
2. Structural patterns
3. Behavioral patterns
All the pattern fall into these three categories. Some of them are listed below as shown:-
- factory pattern
- abstract factory pattern
- strategy pattern
- bridge pattern
- facade pattern
- singleton pattern
- composite pattern
- decorator pattern
- observer pattern
- template pattern
- adapter pattern
Factory Pattern
Factory pattern is one of the most used pattern in java.This type of design pattern comes under creational pattern.This type of pattern provide one of the best way to create an objects.
IMPLEMENTATION
we are trying to create shape interface and concrete classes implementing the shape interface.
A factory class ShapeFactory is defined as a next step.
FactoryPatternDemo ,our demo class will use ShapeFactory to get a Shape object.It will pass information(CIRCLE/RECTANGLE/SQUARE) to ShapeFactory to get the type of object it needs.

Abstract Factory pattern
Abstract factory pattern work around the super factory which creates other factories.This factory is also called as factory of factories.It comes under the creational pattern, that create the object in the best ways.
Implementation
We are trying to create a Shape and Color interface and concrete classes implementing these interface.We creates an abstract factory class AbstractFactory as a next step.Factory classes ShapeFactory and ColorFactory are defined where each factory extend AbstractFactory. A factory creator/generator class FactoryProducer is created.
AbstractFactoryPatternDemo, our demo class uses FactoryProducer to get a AbstractFactory object. It will pass information (CIRCLE/RECTANGLE/SQUARE for shape) to AbstractFactory to get the type of object it needs. It also passes information (RED/GREEN/BLUE for color) to AbstractFactory to get the type of object it needs.
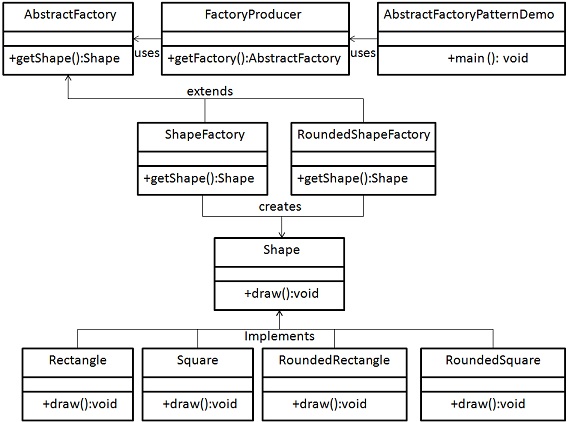
Adapter pattern
Adapter pattern works as a bridge between two incompatible interface. This type of design pattern comes under structural pattern because this pattern combines the capability of two independent interfaces.
This pattern involves a single class which is responsible to join functionalities of independent or incompatible interfaces. A real life example could be a case of card reader which acts as an adapter between memory card and a laptop. you plugins the memory card into card reader and card reader into laptop so that memory card can be read via laptop.
we are demonstrating use of adapter pattern via following example in which an audio player device can play mp3 files only and wants to use an advanced audio player capable of playing vlc and mp4 files.
IMPLEMENTATION
We have an interface MediaPlayer interface and a concrete class AudioPlayer implementing the MediaPlayer interface.AudioPlayer can play mp3 audio files by default.
We have another interface AdvancedMediaPlayer nad concrete classes implementing the AdvancedMediaPlayer interface.these calsses can play vlc and mp4 fprmat files.
We want to make audioPlayer to play other format as well. For this wehave created an adapter class MediaAdapter which implement the MediaPlayer interface and uses AdvancedMediaPlayer objects to play the required format.
AudioPlayer uses the adapter class MediaAdapter passing it the desired audio type without knowing the actual class which can play desired format. AdapterPatternDemo , our demo class will use AudioPlayer class to play various formats.
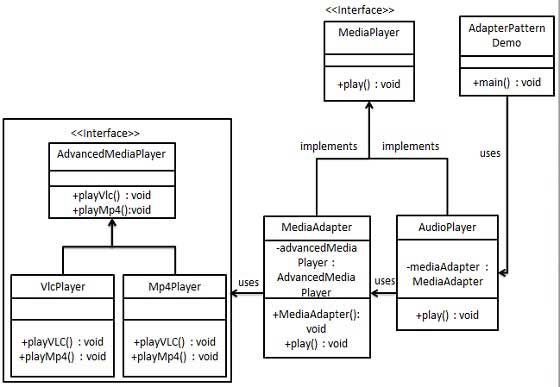
Create interface for Media Player and Advanced Media Player
Mediaplayer.java
public interface MediaPlayer {
public void play(String audioType, String fileName);
AdvancedMediaPlayer.java
public interface AdvancedMediaPlayer { public void playVlc(String fileName); public void playMp4(String fileName); }
Create concrete classes implementing the AdvanceMediaPlayer interface.
vlcPlayer.java
public class VlcPlayer implements AdvancedMediaPlayer{ @Override public void playVlc(String fileName) { System.out.println("Playing vlc file. Name: "+ fileName); } @Override public void playMp4(String fileName) { //do nothing } }
Mp4Player.java
public class Mp4Player implements AdvancedMediaPlayer{ @Override public void playVlc(String fileName) { //do nothing } @Override public void playMp4(String fileName) { System.out.println("Playing mp4 file. Name: "+ fileName); } }
Create adapter class implementing the Mediaplayer interface
MediaAdapter.java
public class MediaAdapter implements MediaPlayer { AdvancedMediaPlayer advancedMusicPlayer; public MediaAdapter(String audioType){ if(audioType.equalsIgnoreCase("vlc") ){ advancedMusicPlayer = new VlcPlayer(); } else if (audioType.equalsIgnoreCase("mp4")){ advancedMusicPlayer = new Mp4Player(); } } @Override public void play(String audioType, String fileName) { if(audioType.equalsIgnoreCase("vlc")){ advancedMusicPlayer.playVlc(fileName); }else if(audioType.equalsIgnoreCase("mp4")){ advancedMusicPlayer.playMp4(fileName); } } }
Create concrete class implementing the MediaPlayer interface.
AudioPlayer.java
public class AudioPlayer implements MediaPlayer { MediaAdapter mediaAdapter; @Override public void play(String audioType, String fileName) { //inbuilt support to play mp3 music files if(audioType.equalsIgnoreCase("mp3")){ System.out.println("Playing mp3 file. Name: "+ fileName); } //mediaAdapter is providing support to play other file formats else if(audioType.equalsIgnoreCase("vlc") || audioType.equalsIgnoreCase("mp4")){ mediaAdapter = new MediaAdapter(audioType); mediaAdapter.play(audioType, fileName); } else{ System.out.println("Invalid media. "+ audioType + " format not supported"); } } }
Use the AudioPlayer to play different types of audio formats.
AdapterPatternDemo.java
public class AdapterPatternDemo { public static void main(String[] args) { AudioPlayer audioPlayer = new AudioPlayer(); audioPlayer.play("mp3", "beyond the horizon.mp3"); audioPlayer.play("mp4", "alone.mp4"); audioPlayer.play("vlc", "far far away.vlc"); audioPlayer.play("avi", "mind me.avi"); } }
Verify the output
Playing mp3 file. Name: beyond the horizon.mp3 Playing mp4 file. Name: alone.mp4 Playing vlc file. Name: far far away.vlc Invalid media. avi format not supported
Decorator Pattern
Decorator pattern allows to add new functionality an existing object without altering its structure. This type of design pattern comes under structural pattern as this pattern acts as a wrapper to existing class.
This pattern creates a decorator class which wraps the original class and provides additional functionality keeping class methods signature intact.
We have trying to explain Decorator pattern using Drawing Editor.
Suppose our drawing editor allows us to include many sorts of shapes including rectangles, ellipses,text,equation,picture etc.
Now we want to introduce a facility into the editor to allow frames to be added to arbitrary objects. For example we might want to put a picture frame around an images, or we might want to frame an equation or some text in a simple box.
IMPLEMENTATION
Since we want to be able to add frames to objects of all types, we could add an attribute into the shape class to specify the type of frame that object has
Pros: - simple and adequate for case where we only
want to add one special attribute to shapes
Cons: - wastes storage since all objects contain all attribute
data the code itself will become clumsy since,
for example,
the draw method will need to have a case switch
for each of the possible frame types
void Shape::draw() {
switch(frame_type) {
case NONE:
break;
case SIMPLE_FRAME:
draw_simple_frame();
break;
...
}
}
void Text::draw() {
Shape::draw();
// render text
}
An alternative would be to derive new classes such as Fr Rectangle,
Fr Picture, Fr Equation etc. to provide framed versions of
each shape class:
Pros: - framing can be restricted to particular shapes
- efficient use of storage since frame data is
only allocated when actually needed
Cons: - huge proliferation in classes
- hard to turn decorations on and off at run-time
Note that the framed versions will inherit exactly the same
interface as their parents. This is important since it is essential that any client using any shape object sees an identical interface.
Singleton Pattern
It's the pattern of single service but multiple user like: there's one receptionist at a hotel and there are multiple visitors. There is one president in a country and million citizens to manage.
Just think of the abstract Vehicle factory that can produce any cars. And there are real vehicle Factory like one id based in Germany (Audi and Mercedes) or factory is based in Italy like Ferrari and Iveco.
The same type of products are created by different factories give different result in the end.
Factory Method Pattern
The factory method is much like Abstract Factory: to create different objects . But the diffidence is that Factory Method is not based on the different Factories but to base on different parameters passed to the creator.
Chain of Responsibility Pattern
The Chain of Responsibility pattern avoids coupling the sender of a request to the receiver , by giving more than one object a chance to handle the request. Mechanical coin sorting bank uses the chain of Responsibility . Rather than having a separate slot for each coin denomination coupled with receptacle for the denomination , a single slot is used . when the coin is dropped , the coin is routed to the appropriate receptacle by the mechanisms within the bank.